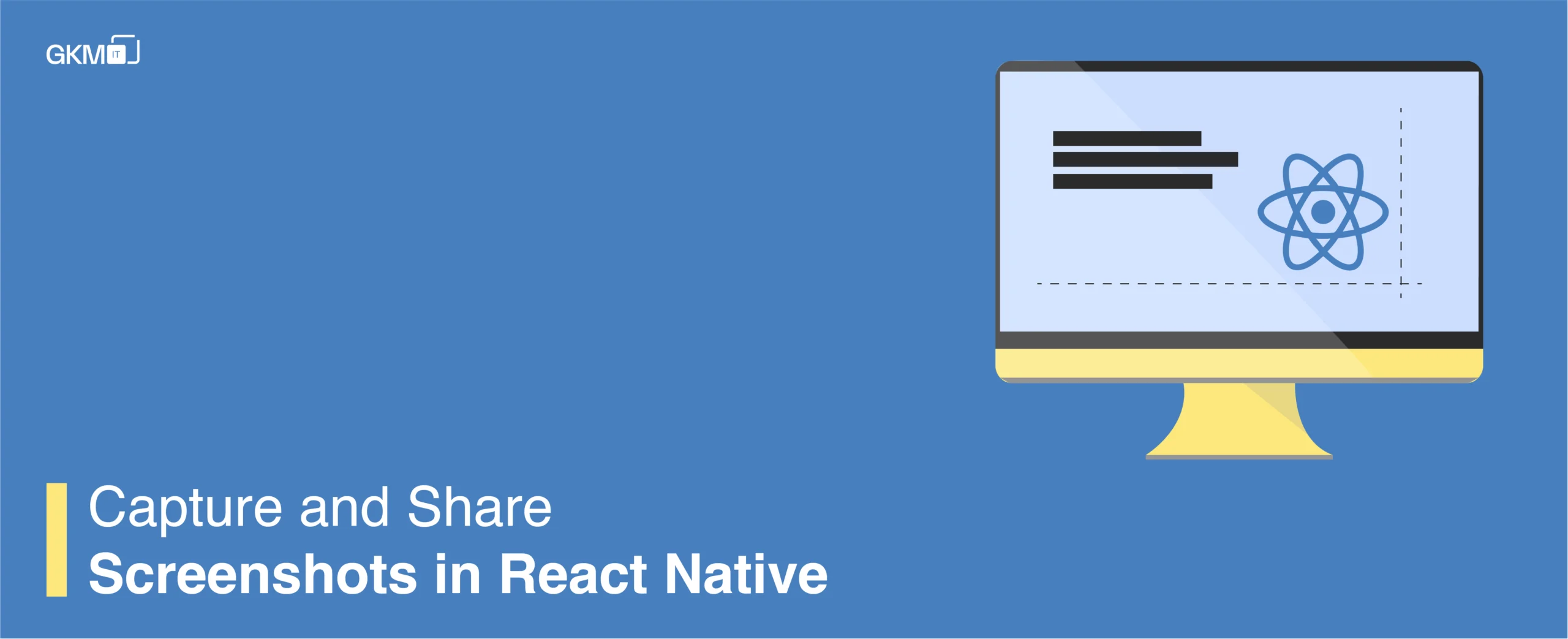
Capture and Share Screenshots in React Native
Many applications require sharing screenshots of the app to other users using different media platforms. Sharing a screenshot in react native is also very easy. Thanks to react-native-view-shot to capture the app’s screen and convert it into an image to share, react-native-fs to access native file systems on the device and react-native-share to share text or image via multiple social media platforms. In this blog, let’s learn how to capture a screenshot and share it.
1. Downloading Dependencies:
yarn add react-native-view-shotyarn add react-native-shareyarn add react-native-fs
Before React Native 0.60.x we would need to link it using the following command:
react-native link react-native-view-shotreact-native link react-native-sharereact-native link react-native-fs
After React Native 0.60.x auto-link should just work.
On iOS we would need to install dependency using pod files:
In the iOS folder of the project, we need to run below command –
npx pod install
2. Capturing Screenshot:
To capture a screenshot of a View, we should make ViewShot as the parent of that View.
Add below line in the import statements:
import ViewShot from ‘react-native-view-shot’;
Update code by making ViewShot as the parent of the View.
<ViewShot style={styles.container} ref="viewShot" options={{format: 'jpg', quality: 0.9}}> <View> <Button style={styles.label} title="Capture and Share" onPress={this.captureAndShareScreenshot} /> </View></ViewShot>
Capture screenshot on button click like –
captureAndShareScreenshot = () => { this.refs.viewShot.capture().then((uri) => { RNFS.readFile(uri, 'base64').then((res) => { let urlString = 'data:image/jpeg;base64,' + res; let options = { title: 'Share Title', message: 'Share Message', url: urlString, type: 'image/jpeg', }; Share.open(options) .then((res) => { console.log(res); }) .catch((err) => { err && console.log(err); }); }); }); };
Conclusion:
See how easy peasy it is to capture screenshot and convert it into an image and share. I hope this blog sound informative and useful to you. If you have any queries, please feel free to contact me on [email protected] or you can drop a message in the comments section below.