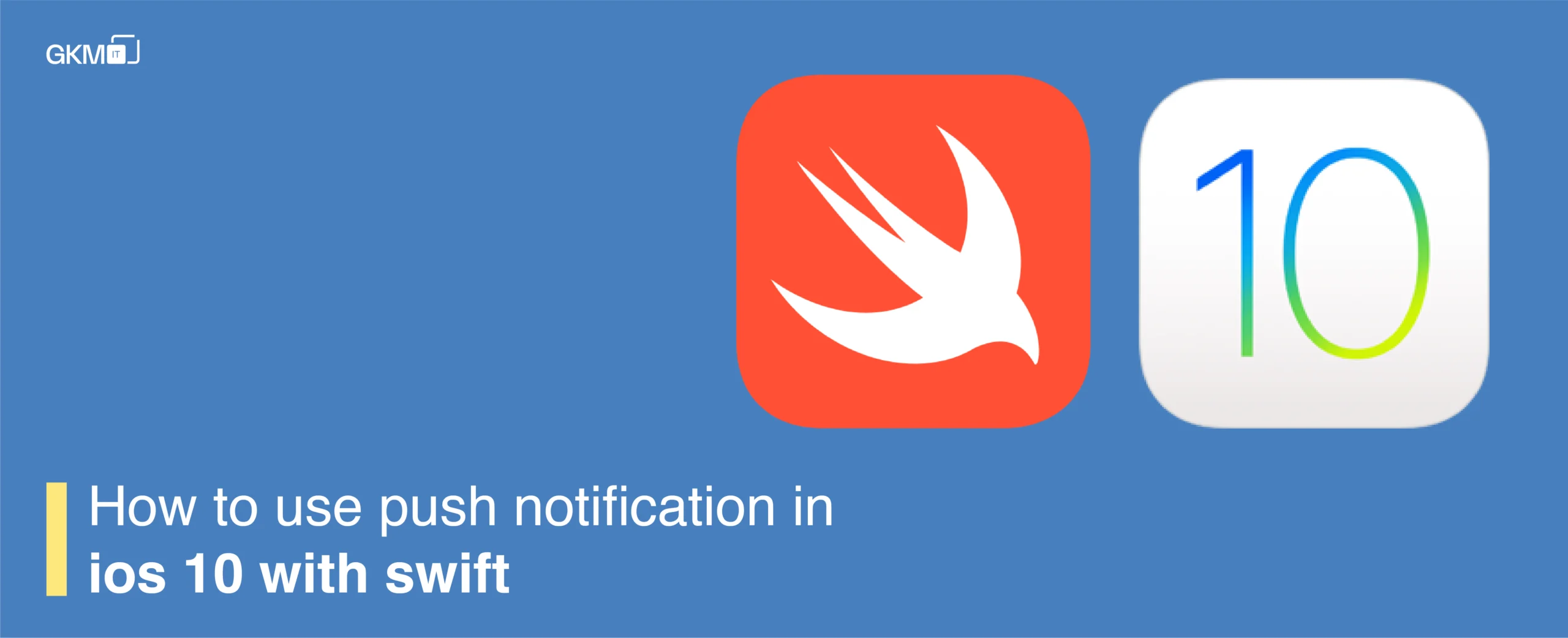
How to use push notification in ios 10 with swift
UserNotifications Framework in iOS 10
In iOS 10, Apple introduced the UserNotifications framework, allowing developers to create rich notifications that include images, sounds, videos, or even custom content generated on the device. This advancement significantly enhances user engagement. Much of this capability comes via three new features showcased at WWDC: media attachments, notification service extensions, and notification content extensions.
Understanding the UserNotifications Framework
The UserNotifications framework (UserNotifications.framework) supports the delivery and handling of local and remote notifications. It allows apps to schedule local notifications based on specific conditions such as time or location. Additionally, apps and extensions can receive and modify local and remote notifications when they are delivered to the userโs device.
Key Features and Classes
The framework provides several important classes that help manage notifications:
- UNCalendarNotificationTrigger โ Triggers a notification at a specific date and time.
- UNLocationNotificationTrigger โ Triggers a notification when the user reaches a specified geographic location.
- UNMutableNotificationContent โ Allows modification of a notificationโs content.
- UNNotification โ Contains the data for a delivered notification.
- UNNotificationAttachment โ Manages media content associated with a notification.
- UNNotificationServiceExtension โ Modifies the content of remote notifications before delivery.
- UNNotificationTrigger โ Provides common behavior for subclasses that trigger notifications.
- UNUserNotificationCenter โ Manages the notification-related activities for your app.
Implementing Push Notifications in iOS 10
Step 1: Set Up Certificates
Log in to your Apple Developer Account, create the necessary certificates, and ensure that Push Notification Services are enabled.
Step 2: Create a New Xcode Project
Set up a new project in Xcode and configure it correctly.
Step 3: Configure Target Settings
Navigate to Targets โ General, select your Team, or choose a valid provisioning profile. Ensure that your Bundle Identifier matches the one in your certificate.
Step 4: Enable Push Notifications
Go to Capabilities and enable Push Notifications.
Step 5: Modify AppDelegate.swift
Import the UserNotifications framework and implement UNUserNotificationCenterDelegate in your AppDelegate
class.
import UserNotifications
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
registerForPushNotifications(application)
return true
}
func registerForPushNotifications(_ application: UIApplication) {
UNUserNotificationCenter.current().delegate = self
UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { granted, error in
if granted {
DispatchQueue.main.async {
application.registerForRemoteNotifications()
}
}
}
}
func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
print("Failed to register for remote notifications: \(error.localizedDescription)")
}
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
let tokenString = deviceToken.map { String(format: "%02.2hhx", $0) }.joined()
print("Device Token: \(tokenString)")
}
Step 6: Test Push Notifications
After completing the setup, use APN Tester to send a push notification.
Why Push Notifications Matter in Mobile App Development
Push notifications play a crucial role in modern Mobile App Development Services by enhancing user engagement and retention. They allow businesses to send timely updates, promotional offers, and reminders to users, ensuring a seamless interaction with the app. Implementing Mobile App Development Service strategies that leverage push notifications effectively can significantly improve user experience.
Conclusion
With the UserNotifications framework, Apple has provided developers with a robust toolset to create rich and interactive notifications. By following the steps above, you can integrate push notifications into your iOS app and ensure a seamless experience for your users.
Try implementing push notifications in your next project and explore the possibilities they bring!